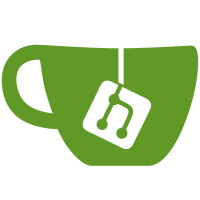
Now InputTranslator has its own interface to provide polymorphism in GameSession class Touch input read only if target platform is android
36 lines
795 B
C#
36 lines
795 B
C#
using UnityEngine;
|
|
|
|
public class KeyBinding : IBinding
|
|
{
|
|
private KeyCode keyBinding;
|
|
private KeyCode alternativeKeyBinding;
|
|
public bool IsRestricted { get; set; }
|
|
|
|
public KeyBinding(KeyCode key,KeyCode alternative = KeyCode.None)
|
|
{
|
|
keyBinding = key;
|
|
alternativeKeyBinding = alternative;
|
|
IsRestricted = false;
|
|
}
|
|
|
|
public void UpdateBinding(KeyCode key)
|
|
{
|
|
keyBinding = key;
|
|
}
|
|
public void UpdateAlternativeBinding(KeyCode key)
|
|
{
|
|
keyBinding = key;
|
|
}
|
|
|
|
public bool IsReleased()
|
|
{
|
|
return Input.GetKeyUp(keyBinding) || Input.GetKeyUp(alternativeKeyBinding);
|
|
}
|
|
|
|
public bool IsPressed()
|
|
{
|
|
return Input.GetKeyDown(keyBinding) || Input.GetKeyDown(alternativeKeyBinding);
|
|
}
|
|
|
|
}
|